Friday, November 27, 2009
Using BFS to solve the minimum number moves problems
Eg.
1
/ \
2 3
/ \ / \
4 5 6 7
For a tree like the above, a BFS traversal guarantees the traversal order: 1->2->3->4->5->6->7
Though in a linked implementation of a tree, the BFS traversal is a bit trickier but for an array representation this is nothing but a straight traversal of the array sequentially.
Since in most of the programming problems, generally the tree is not known at the beginning, therefore the limit of the total number of nodes is uncertain. But, theres nothing to worry much, for the ArrayList in Java and vector classe in C++ are the way out. Well a generalized BFS traversal may look something like this, when the code to remove the repeated states is added to the main BFS logic:
(Note: its just a pseudo code)
fringe= a queue
closed= a set containing visited nodes
fringe.push(root_node_of_tree);
while( ! fringe.empty())
{
node n=fringe.pop();
if(goalTest(n)){
return solution(n);
}
if(!closed.contains(n)){
closed.add(n);
//add all children of n to fringe
}
}
Based on this below is my C++ implementation for finding out the minimum move sequence to solve the 8-puzzle problem. In this problem given a 3*3 matrix with numbers 1..8 filled in random 8 places among 9 in the matrix with 1 block empty. Our goal is to slide a block at a time in the empty place and finally arrange the puzzle back to sorted order, just like this
1 2 3
4 5 6
7 8 0
(Note: 0 indicates the empty block)
Here is my complete C++ implementation
Sunday, November 8, 2009
An Effective way of removing duplicates in a file
Well while surfing internet, I later found that the tree concept I used to solve this problem is pretty much similar to a standard implementation called 'Suffix tree'. Here I am not going to explain you about suffix trees, you can find plenty of matter on this once you google it, rather, I would be explaining how my solution to this problem worked. Well, I don't claim it to be the most optimal implementation, I am sure, someone out there may come up with a solution better than mine. But this one solves the purpose just fine.
The logic here is to keep building the custom tree (which now we know is similar to suffix tree) as we go through the text. Here is the basic algorithm:
1) Iterate through the text and do the following steps
1.1) As the beginning of a new word is encountered, start matching it starting from the root node to its children, successively. along with buffering the current word as we proceed.
1.2) If a match is found, try to match the next character in the word with its children
1.3) If a match is not found, add another node representing the current character as a child to the current node.
1.4) When the word ends, and the last character is matched, check its 'final' tag.
1.4.1) if its true, it means the current word is the repeated one, so don't output the current buffer word
1.4.2) if its false, it means the current word is encountered for the first time, so output the current buffer and flush the buffer.
Eg. let the text be "CAT CAN BAT". The tree for this sentence will be -
ROOT
/\
C B
/ \
A A
/ \ \
T* N* T*
Note: the (*) mark represents the final state.
Here goes my C/C++ implementation
Sunday, October 25, 2009
Saturday, October 24, 2009
Dealing with a Strange Java Problem
interface test {
void fun();
}
public class Test implements test {
public void fun() {
System.out.println("Test.fun() called");
}
public static void main(String[] args) {
new Test().fun();
}
}
Can you find any problem with the above code sample? Well there really isn't any problem with the above code. Still when you try to run this on Windows systems the execution fails for Test class ( i.e. >java Test) throwing exceptions like:-
java.lang.NoClassDefFoundError: test (wrong name: Test)
at java.lang.ClassLoader.defineClass1(Native Method)
at java.security.AccessController.doPrivileged(Native Method)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:276)
at java.lang.ClassLoader.defineClass1(Native Method)
at java.security.AccessController.doPrivileged(Native Method)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:276)
Exception in thread "main"
Exception in thread "main" Java Result: 1
And adding to the surprise, this program runs absolutely fine on Linux and other Unix based systems!
Ok so lets try to dissect the program and figure out what the problem is with this. Here is the possible explaination which I came up with.
Observing it a little carefully you can observe that the name of the interface is 'test' and class is 'Test' this makes them differ only in case. But what difference does it make to us? java is of course case sensitive, so we are free to chose names like that!
Well the answer is that, although java is case sensitive the underlying OS ( Windows) is not. Therefore the compiler (even though it wanted) couldn't create two separate .class files (one for interface, and other for the implementing class), because the other class file over-writes the previous one. In short, this problem arises because after compiling the above code, two class files: test.class and Test.class map only to a single file on windows, not two unlike the unix systems.
So be careful while getting trapped in such of hard-to-find bugs!
Saturday, September 5, 2009
Getting started with GNOME based OS (Linux)

Thats the beauty of open-source- it can give you exactly what you want. Open-source products including Linux opens itself to change by anyone who uses it thereby giving the freedom and power to users. There are multiple Window Managers available for using with Linux. The most commonly used are -GNOME and KDE which generally comes integrated with most of the linux distributions.
Lets begin with our familiarisation with GNOME, Ubuntu9.04 Desktop ( but this might help you in other distros as well)
It displays a menu bar at the top containing menus for Application, Places and System on the left side of the bar along with the tray icons of the processes and a calendar, sound control and the Username being on the right side of the bar.
And a status bar at the bottom of the screen with the icon to Show the empty desktop minimizing all the currently opened windows, tabs of all the currently opened windows, a Desktop Switcher showing the available desktops and finally a Trash (where the deleted files reside giving option to restore back the accidently deleted files, until manually cleaned).
- Application Menu: It contains Set of the applications installed and currently configured to be displayed in the menu format in different categories. It comes packed with a range of open-source applications preinstalled, like firefox for web browsing, Evolution as the email client, Pidgin as the chatting messenger, Totem and Rythmboxfor audio/video playing, GIMP ( my favourite!) for Image editing and a set of GNOME games!
- Places Menu: It presents links to some of the predefined locations so as to reach there quickly.
- System Menu: It presents the system specific tasks and configurations
Application and System menu can be manually altered by right clicking-> Edit Menu option.
Any system related information about the running processes or the present system memory and network usage etc. can be found anytime in System->Administration->System Monitor.
Ubuntu presents with an exhaustive list of open-source software for anyone to download from anywhere in the world from its central repository. This can be done in either of the following ways:
- Applications->Add/Remove
- System->Administration->Synaptic Package Manager
- using command line utilities like apt-get :
- sudo apt-get install
Some of the other utilities in which you would be interested to install are:
- Opera (a web browser)
- Netbeans IDE ( for programming in JavaSE, JavaME, JavaEE, C, C++, Javascript etc )
- openssh ( for enabling remote login into the machine)
- Thunderbird ( another email client like Evolution)
- Avant Window Navigator ( enables a cool looking, highly customizable docking feature much like MAC )
- GVIM text editor ( a GUI based vim text editor, for the people who are addicted to the cool and simple, our beloved VIM ! )
Happy Linuxing!
Saturday, August 22, 2009
Mother Nature
Here are the lines written by a 15 year school girl. It indeed reminds us of our long forgotten nature and provokes us to again ponder on our actions.
Mother Nature
The lap of soothing comforts for all
With selfless care for all
Where all worries vanish, with no blemish,
Where the delicate colours of the rainbow,
Stretch themselves in the sky,
Birds sing, high and higher they fly.
Trees bear sweet fruits of affection,
Rivers linger through cresses with anticipation
This, nature through its humble phase,
confers on man a graceful place.
Man today in his passionate greed,
cutting down trees without need,
The smoke looming high from chimneys,
Toxicants thrown into rivers by industries.
Exploring, exploiting, denuding, eroding...
Man is gradually destroying his own living.
Benumbing, choking the Mother Nature.
Forgetting all her cares and nurture.
Tomorrow someone will surely ask:
Who is Mother Nature?
The barren lands with no life?
Or the carcasses of early extinct animals?
Is it???
So, can't we be kinder to nature,
for all her care and nurture?
can't we strive today,
So that never comes this tomorrow.
So that Mother Nature never cease to grow.
Can't We???
--Anjali Sharma
( fortunately, who is my beloved sis )
GIMP (the GNU Image Manipulation Program) my favourite Open-Source Image Editor
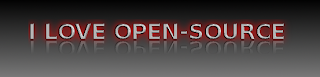
Here are the easy steps to create one-
1. Install and start GIMP editor in your favourite operating system. This is fairly easy in case of OpenSolaris and Linux flavours like Ubuntu, Fedora etc.
2. File -> New -> Choose desired size ( default 640*400 works fine for me ), and click OK
Now a white background is visible in the Image window.
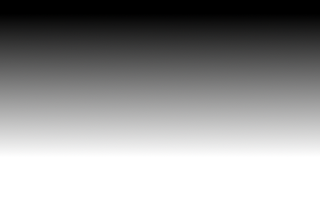
3. Select Blend Tool and Gradient as FG to BG. Then keeping the CTRL key pressed drag a line from top to bottom on the image and you shall find something like this -->
4. Select the Text tool from Toolbar or press 'T' key shortcut. and drag a bounding rectangle on the image at the position you want to place the text. This will open a text box popup, type in the de
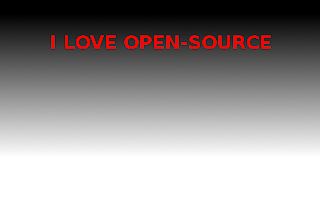
5. Right click on the newly created layer with the name same as your text and select Duplicate Layer . This creates a duplicated layer ( say Layer2). Now with the original layer, Layer1 selected, go to
Filters -> Blur -> Gaussian Blur -> Select horizontal and vertical blur radius as anything between 12 px - 20px each. and click OK
6. Select Layer2 and choose Color as Green from the color chooser.
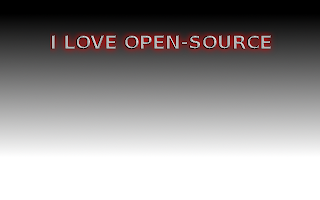
7. Select Filters-> Distorts -> Emboss -> select funtion as Emboss and Azimuth, Elevation and Depth to any suitable value ( 30, 45, 20 respectively in my case)
now this will look something like this->
8. Select Layer2 -> right click on it -> Duplicate Layer. This creates another duplicate layer of it ( say Layer3).
9. Now Select Layer3 -> Tools menu -> Transform Tools -
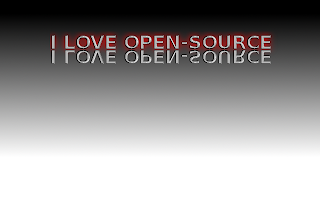
Select the Move tool from the toolbar and position the vertically flipped text few pixels below the original text. It will look like this now--->
10. Select Layer3 -> right click on it -> Add Layer Mask -> select
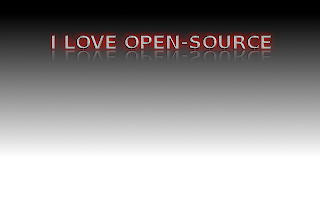
11. Select Layer3 and then select Blend tool from the tool bar with Gradient as FG to transparent and using CTRL key draw a straight line from bottom to top anywhere in the image. This is make the reflection text only partially visible, like this->
12. Finally right click Layer3 -> Apply Layer Mask. This finishes our steps and we finally get the desired text, kept on a shiny table, and glowing in red light. Crop the image to remove unwanted space and thats all!
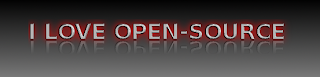
Hope you enjoyed the tutorial! Experiment and learn...
Tuesday, August 11, 2009
Yet another tremor shook the souls at Bhubaneswar.
These increasing terrorist activities, bomb blasts every now and then, and now earth-quakes, tsunami, drastic weather changes, global warming, pollution, Swine Flu! are they not signals by mother nature indicating something? It sounds like a threat to me. We all shall be wiped off from the crust if we continued our greedy actions..
But at such hard times, one deeply feels the need of the loved ones to stay next to us. It makes one realise the true worth of relationships.
Saturday, August 8, 2009
A Leap towards Open Source... We too are in the race !
I really appreciate this campaign and am happy to be a part of this :) . I hope, soon I would be accompanied by all my fellow mates in this effort.
Monday, August 3, 2009
My Experiments with Java..
Java... I remember I heard it for the first time in class 7th from my computer teacher. I didnt know anything more than just its name. At that time computers meant no more than a dull boring Black screens placed in our school labs, which knows some DOS commands, for me. Java seemed interesting.. :) or rather 'sounded' interesting! I decided to learn it sooner or later. But then we make so many plans in our lives when we are young. And as it happens with me always, I forgot even that. It wasnt until my B-Tech 1st year, that again the term 'Java' got refreshed in my mind. Actually it was an informal geeky (rather we all were trying to sound as geeky as possible!) conversations among friends at college hostel. There one of my dear friend put up this term & again I was reminded of my plans of childhood :) (sounds comical)
Well then it was the summer holidays of 2nd year and then I decided to try my hands out on something new. And what else can be it other than Java at that time. I just dived my college library and came out satisfied with a thich 1000 pages book on Advanced Java in hands. I happily took it home and then spent 2-3 days just to understand its starting 2-3 pages. :) It began with Multi-threading !!! that was the first topic I started with!
anyways.. it happens.. I kept on trying and consistently practicing all the programs and soon I had a large collection of my Java programs and gradually the 4 letters of 'Java' started getting clearer in my mind. And just few months more and I was the champ in Java among our friends !! ( i know I boast sometimes ) Another friend Raju, who started with C# at nearly the same time when I picked up Java, too started making cool applications in it. We used to spend hours together competing with each other, challenging each other on programming problems. He used to do it in C# and by now you all very well know what language I used:) ... This is called a healthy beginning. Later I self-studied JavaME an JavaEE, created applications ranging from stupid games to challenging problems. Here are few of my startup programs in Java. freely available and distributable, thats what open-source is all about! -
Saturday, August 1, 2009
Another KIIT-Sun Club get-together
It was only this Saturday ie 21st March'09 and the KIIT- Sun Club was all set to organize a Technical demo on OpenSolaris2008.11.
It was primarily focused for the 1st and 2nd year students who do not know the intricacies of the operating systems and the specialities of OpenSolaris, in order to equip them with the basic knowledge of OpenSolaris Operating System, but was equally open for interested students of all the years and branches. And to my surprize I got the students for the 3rd years also joining me in the talk and few were found much interested to know the correlation between modern Linux and opensolaris as they look alike to them.
I started with the history of opensolaris project and later on introduced them with the tools to be used late in that session i.e. Virtual Box. Smart IT students didn't take it any longer to understand the underlying basics and working of Virtual Box as most of them have already seen some kind of Virtualization agents earlier. Few seemed to be already using Virtual Box, but still they liked to listen to the basics of the concept of Virtualization. Testing entirely different and new operating systems on their Windows preloded systems provided by the college, have never been so easy and risk free for them. Watching the Guest operating system run inside the host machine was though some kind of a magic to few but nonetheless all were keen to know the steps to do it. After covering the basics I demonstrated the steps for building Virtual Machines inside Virtual Box and how can the guest OS be installed in it safely.
I later showed them Opensolaris working perfectly fine in the Virtual Box ( preinstalled on my computer). Then after giving them a brief overview of the opensolaris I talked about the exciting features and opensolaris like DTrace and promised them to carry on this topic in future sessions.
Friday, May 1, 2009
Its hot !
and I am feeling this place.. more like a cage..
this place will always be, the 1 which anyone would like to flee
But this hostel life has not really been totally wasteful
now look here we are taught so many good lessons-
> The calm and cool Birthday celebrations ( i hope u get it.) taught us the lesson of non-violence and brotherhood as never before.. The way we guys congratulate and greet others on their B'days ( well its the time which is much more awaited by all other than only d b'day boy ) .. m talkin abt d birthday bumps yar.. its way of living in a society. It shows the existence of immense intimacy between guys of King's Palace-3 . yeah thats what true friendship seems to me!
> The delicious food ( Oh Paneer!!! yummy. look I started developing a taste for it ). It teaches us to respect the food and be thankful to hostels mess authorities for the little we are getting in our hostels ( mind the word 'little') :)
as such paneer is the only source of energy here for vegetarians like me
> Watching movies even a day before exams doesnt really teach us anything but its just to tell that we are sharp enough to vomit in few tragic hours of exams all what in entire semester's class we never thought of giving an ear to.
Anyways its true that theres a beautiful dawn awaiting after a gloomy night.
> Games !!! oh yes. playing it whole night really helps a lot in building ones concentration and keeping ones mind from silly thoughts and earthly tensions. its a divine thing to do. and yes ofcourse it keeps your laptops from rusting.
I really feel everyone should atleast ones experience this hostel life, full of fun . among all this you really do discover some true friends with whom you would like to hang out ( else they are always ready to Hang you out and then get themselves hung too). u rarely find such idiots!
Friday, March 6, 2009
Netbeans Technical session
Since the audience I targeted were mostly second year students well trained in their curriculum subjects like C++ and Operating Systems but having little or no background knowledge of Java, but were much enthusiastic to learn it. So, I planned to show them how easily and quickly can the projects be developed using Netbeans without the need of having a strong command on the language.
I began by telling them how easy and fun would C++ programming be in Netbeans, and hence can be used for their daily assignments. I introduced Netbeans as the IDE which they shall be using exhaustively in most of their future projects. Bundled with JavaSE,JavaEE, JavaME, JavaScript, Ruby, application and database servers and much more, Netbeans offers support for just anything one can think of!
I developed a sample project each in JavaSE, JavaEE and JavaME by typing down minimum number of Java Code lines by just using other features of Netbeans like drag and drop etc. trying to keep the complexity of the project minimum, so that it can be easily understood by the audience.
Students were really fascinated to see ease of development it offers in Mobile application in JavaME, after watching the development of a 'Login and Welcome' application in which I just typed in a single line of Java Code!
Everyone was willing to adapt Netbeans as their IDE at the end of the session.
At the end their was a usual quiz session with exciting goodies to be won. Netbeans DVDs were distributed among the Club members so than they can experiment, play and learn.
Think anything Code anything
Wednesday, February 25, 2009
My first ever Session...
Last Sunday I conducted a little casual type of OSUM meeting just to brief up the interested students (especially of 2nd years) about SUN, its technologies, OSUM, SAI and the benefits its going 2 share with us. I got a mass of interested students (more than 50) attending the session (45 mins approximately) willingly!
I got amazed to know that though majority of them were not knowing of Java Programming

(since it was not in their course till now) but they had much enthusiasm to learn it and go for the Certifications as well!!!
Opposite to what I expected and adding to my astonishment, there were 5-6 people who came forward showing their extreme interest in going for SUN Certification in near future and are eager to derive benefits out of SAI !
Many were curious to know about various SUN Technologies which I assured them to be discussed in future OSUM meetings .

At the end I had a brief quiz. I asked few questions to them and the ones who came up with correct answer got SUN goodies ( Open Solaris Student pack, Netbeans DVD, SUN pen, SUN keyrings etc.)
Since it was my first demo I ever conducted. I was a bit nervous about it and a bit uncertain about the response. But it all went so well. Thus making me to think an urgent need of other such demos. I think I would be conducting another session very soon.
It boosted my confidence a lot... A nice and exciting experience altogether..
Saturday, January 24, 2009
truly OSUM (awesome) !!!

Here is a community in which you might be interested. OSUM (pronounced "awesome") is a global community of students that are passionate about Free and Open Source Software (FOSS) and how it is Changing (Y)Our World.
OSUMs provide students with training in cutting edge technologies in demand by the IT industry, access to resources to advance their careers and the opportunity to make friends through engaging and fun technology-based activities. Students will reap substantial benefits which include-
- Support for FOSS ( Free and Open Source Softwares)
- Hands- on training via Tech Demos and Student Projects to learn technologies that can lead to promising careers in the IT industry
- Tools, ideas and opportunity for student projects, contests and more
- Ready access to free and low-cost student resources, such as the SAI (free trainng and discounted certification)
- SAI offers loads of tutorials on all the SUN technologys
- A HUGE discount !!! on SUN Certification exams ( $40 instead of $200)
- free ePractice exams for Sun Certification preparation and much more
- Communities create opportunity for collaboration
- Make lots of like-minded (technical) friends through the Sun OSUM global community
- Have access to a variety of OSUM events such as Software Freedom Day, University Day, etc.
- Media kits & Give-aways
you can find me here.
be a part of KIIT-U SUN club here.
Feel free to tell about this wonderful club to all your friends.
Participate in Project NPTEL
In the true spirit of being 'open' , volunteers are solicited to help to transcribe the video lectures into text.
Of course, due recognition will be awarded.
IIT Madras' NPTEL project is rated 4th among the world's e-learning
intiatives & this is a great way to contribute and make it even better.
The IIT Madras has made video lectures of all IITs & IISc professors' lectures on various subjects, for details http://nptel.iitm.ac.in/ or http://in.youtube.com/user/
Now they want to transcribe them into written format. So, our task will be, to listen the video lectures & write down whatever the professor says.
This is beneficial for you anyway, as you'll be getting the material from top professors of IIT & IISc. Computer Science, EC, ME, IT, EE etc all branch's various subjects are covered in this. Getting IIT's quality education is dream of every student, isn't it :-)
Therefore, any branch student who is interested in this, please give their names & email id.So Hurry up friends..
1. Name :
2. Email :
Looking forward to your confirmations to contribute.
Regards,
--
Anurag Sharma
SUN CA, KIIT
(A.Sharma@sun.com)
Wednesday, January 21, 2009
Top Ten Errors Java Programmers Make
(How to spot them. How to fix/prevent them.)
-By David Reilly
Whether you program regularly in Java, and know it like the back of your hand, or whether you're new to the language or a casual programmer, you'll make mistakes. It's natural, it's human, and guess what? You'll more than likely make the same mistakes that others do, over and over again. Here's my top ten list of errors that we all seem to make at one time or another, how to spot them, and how to fix them.
10. Accessing non-static member variables from static methods (such as main)
Many programmers, particularly when first introduced to Java, have problems with accessing member variables from their main method. The method signature for main is marked static - meaning that we don't need to create an instance of the class to invoke the main method. For example, a Java Virtual Machine (JVM) could call the class MyApplication like this :-
MyApplication.main ( command_line_args );
This means, however, that there isn't an instance of MyApplication - it doesn't have any member variables to access! Take for example the following application, which will generate a compiler error message.
public class StaticDemo
{
public String my_member_variable = "somedata";
public static void main (String args[])
{
// Access a non-static member from static method
System.out.println ("This generates a compiler error" +
my_member_variable );
}
}
If you want to access its member variables from a non-static method (like main), you must create an instance of the object. Here's a simple example of how to correctly write code to access non-static member variables, by first creating an instance of the object.
public class NonStaticDemo
{
public String my_member_variable = "somedata";
public static void main (String args[])
{
NonStaticDemo demo = new NonStaticDemo();
// Access member variable of demo
System.out.println ("This WON'T generate an error" +
demo.my_member_variable );
}
}
9. Mistyping the name of a method when overriding
Overriding allows programmers to replace a method's implementation with new code. Overriding is a handy feature, and most OO programmers make heavy use of it. If you use the AWT 1.1 event handling model, you'll often override listener implementations to provide custom functionality. One easy trap to fall into with overriding, is to mistype the method name. If you mistype the name, you're no longer overriding a method - you're creating an entirely new method, but with the same parameter and return type.
public class MyWindowListener extends WindowAdapter {
// This should be WindowClosed
public void WindowClose(WindowEvent e) {
// Exit when user closes window
System.exit(0);
}
});
Compilers won't pick up on this one, and the problem can be quite frustrating to detect. In the past, I've looked at a method, believed that it was being called, and taken ages to spot the problem. The symptom of this error will be that your code isn't being called, or you think the method has skipped over its code. The only way to ever be certain is to add a println statement, to record a message in a log file, or to use good trace debugger (like Visual J++ or Borland JBuilder) and step through line by line. If your method still isn't being called, then it's likely you've mistyped the name.
8. Comparison assignment ( = rather than == )
This is an easy error to make. If you're used other languages before, such as Pascal, you'll realize just how poor a choice this was by the language's designers. In Pascal, for example, we use the := operator for assignment, and leave = for comparison. This looks like a throwback to C/C++, from which Java draws its roots.
Fortunately, even if you don't spot this one by looking at code on the screen, your compiler will. Most commonly, it will report an error message like this : "Can't convert xxx to boolean", where xxx is a Java type that you're assigning instead of comparing.
7. Comparing two objects ( == instead of .equals)
When we use the == operator, we are actually comparing two object references, to see if they point to the same object. We cannot compare, for example, two strings for equality, using the == operator. We must instead use the .equals method, which is a method inherited by all classes from java.lang.Object.
Here's the correct way to compare two strings.
String abc = "abc"; String def = "def";
// Bad way
if ( (abc + def) == "abcdef" )
{
......
}
// Good way
if ( (abc + def).equals("abcdef") )
{
.....
}
6. Confusion over passing by value, and passing by reference
This can be a frustrating problem to diagnose, because when you look at the code, you might be sure that its passing by reference, but find that its actually being passed by value. Java uses both, so you need to understand when you're passing by value, and when you're passing by reference.
When you pass a primitive data type, such as a char, int, float, or double, to a function then you are passing by value. That means that a copy of the data type is duplicated, and passed to the function. If the function chooses to modify that value, it will be modifying the copy only. Once the function finishes, and control is returned to the returning function, the "real" variable will be untouched, and no changes will have been saved. If you need to modify a primitive data type, make it a return value for a function, or wrap it inside an object.
When you pass a Java object, such as an array, a vector, or a string, to a function then you are passing by reference. Yes - a String is actually an object, not a primitive data type. So that means that if you pass an object to a function, you are passing a reference to it, not a duplicate. Any changes you make to the object's member variables will be permanent - which can be either good or bad, depending on whether this was what you intended.
On a side note, since String contains no methods to modify its contents, you might as well be passing by value.
5. Writing blank exception handlers
I know it's very tempting to write blank exception handlers, and to just ignore errors. But if you run into problems, and haven't written any error messages, it becomes almost impossible to find out the cause of the error. Even the simplest exception handler can be of benefit. For example, put a try { .. } catch Exception around your code, to catch ANY type of exception, and print out the message. You don't need to write a custom handler for every exception (though this is still good programming practice). Don't ever leave it blank, or you won't know what's happening.
For example
public static void main(String args[])
{
try {
// Your code goes here..
}
catch (Exception e)
{
System.out.println ("Err - " + e );
}
}
4. Forgetting that Java is zero-indexed
If you've come from a C/C++ background, you may not find this quite as much a problem as those who have used other languages. In Java, arrays are zero-indexed, meaning that the first element's index is actually 0. Confused? Let's look at a quick example.
// Create an array of three strings
String[] strArray = new String[3];
// First element's index is actually 0
strArray[0] = "First string";
// Second element's index is actually 1
strArray[1] = "Second string";
// Final element's index is actually 2
strArray[2] = "Third and final string";
In this example, we have an array of three strings, but to access elements of the array we actually subtract one. Now, if we were to try and access strArray[3], we'd be accessing the fourth element. This will case an ArrayOutOfBoundsException to be thrown - the most obvious sign of forgetting the zero-indexing rule.
Other areas where zero-indexing can get you into trouble is with strings. Suppose you wanted to get a character at a particular offset within a string. Using the String.charAt(int) function you can look this information up - but under Java, the String class is also zero-indexed. That means than the first character is at offset 0, and second at offset 1. You can run into some very frustrating problems unless you are aware of this - particularly if you write applications with heavy string processing. You can be working on the wrong character, and also throw exceptions at run-time. Just like the ArrayOutOfBoundsException, there is a string equivalent. Accessing beyond the bounds of a String will cause a StringIndexOutOfBoundsException to be thrown, as demonstrated by this example.
public class StrDemo
{
public static void main (String args[])
{
String abc = "abc";
System.out.println ("Char at offset 0 : " + abc.charAt(0) );
System.out.println ("Char at offset 1 : " + abc.charAt(1) );
System.out.println ("Char at offset 2 : " + abc.charAt(2) );
// This line should throw a StringIndexOutOfBoundsException
System.out.println ("Char at offset 3 : " + abc.charAt(3) );
}
}
Note too, that zero-indexing doesn't just apply to arrays, or to Strings. Other parts of Java are also indexed, but not always consistently. The java.util.Date, and java.util.Calendar classes start their months with 0, but days start normally with 1. This problem is demonstrated by the following application.
import java.util.Date;
import java.util.Calendar;
public class ZeroIndexedDate
{
public static void main (String args[])
{
// Get today's date
Date today = new Date();
// Print return value of getMonth
System.out.println ("Date.getMonth() returns : " +
today.getMonth());
// Get today's date using a Calendar
Calendar rightNow = Calendar.getInstance();
// Print return value of get ( Calendar.MONTH )
System.out.println ("Calendar.get (month) returns : " +
rightNow.get ( Calendar.MONTH ));
}
}
Zero-indexing is only a problem if you don't realize that its occurring. If you think you're running into a problem, always consult your API documentation.
3. Preventing concurrent access to shared variables by threads
When writing multi-threaded applications, many programmers (myself included) often cut corners, and leave their applications and applets vulnerable to thread conflicts. When two or more threads access the same data concurrently, there exists the possibility (and Murphy's law holding, the probability) that two threads will access or modify the same data at the same time. Don't be fooled into thinking that such problems won't occur on single-threaded processors. While accessing some data (performing a read), your thread may be suspended, and another thread scheduled. It writes its data, which is then overwritten when the first thread makes its changes.
Such problems are not just limited to multi-threaded applications or applets. If you write Java APIs, or JavaBeans, then your code may not be thread-safe. Even if you never write a single application that uses threads, people that use your code WILL. For the sanity of others, if not yourself, you should always take precautions to prevent concurrent access to shared data.
How can this problem be solved? The simplest method is to make your variables private (but you do that already, right?) and to use synchronized accessor methods. Accessor methods allow access to private member variables, but in a controlled manner. Take the following accessor methods, which provide a safe way to change the value of a counter.
public class MyCounter
{
private int count = 0; // count starts at zero
public synchronized void setCount(int amount)
{
count = amount;
}
public synchronized int getCount()
{
return count;
}
}
2. Capitalization errors
This is one of the most frequent errors that we all make. It's so simple to do, and sometimes one can look at an uncapitalized variable or method and still not spot the problem. I myself have often been puzzled by these errors, because I recognize that the method or variable does exist, but don't spot the lack of capitalization.
While there's no silver bullet for detecting this error, you can easily train yourself to make less of them. There's a very simple trick you can learn :-
* all methods and member variables in the Java API begin with lowercase letters
* all methods and member variables use capitalization where a new word begins e.g - getDoubleValue()
If you use this pattern for all of your member variables and classes, and then make a conscious effort to get it right, you can gradually reduce the number of mistakes you'll make. It may take a while, but it can save some serious head scratching in the future.
(drum roll)
And the number one error that Java programmers make !!!!!
1. Null pointers!
Null pointers are one of the most common errors that Java programmers make. Compilers can't check this one for you - it will only surface at runtime, and if you don't discover it, your users certainly will.
When an attempt to access an object is made, and the reference to that object is null, a NullPointerException will be thrown. The cause of null pointers can be varied, but generally it means that either you haven't initialized an object, or you haven't checked the return value of a function.
Many functions return null to indicate an error condition - but unless you check your return values, you'll never know what's happening. Since the cause is an error condition, normal testing may not pick it up - which means that your users will end up discovering the problem for you. If the API function indicates that null may be returned, be sure to check this before using the object reference!
Another cause is where your initialization has been sloppy, or where it is conditional. For example, examine the following code, and see if you can spot the problem.
public static void main(String args[])
{
// Accept up to 3 parameters
String[] list = new String[3];
int index = 0;
while ( (index < args.length) && ( index < 3 ) )
{
list[index++] = args[index];
}
// Check all the parameters
for (int i = 0; i < list.length; i++)
{
if (list[i].equals "-help")
{
// .........
}
else
if (list[i].equals "-cp")
{
// .........
}
// else .....
}
}
This code (while a contrived example), shows a common mistake. Under some circumstances, where the user enters three or more parameters, the code will run fine. If no parameters are entered, you'll get a NullPointerException at runtime. Sometimes your variables (the array of strings) will be initialized, and other times they won't. One easy solution is to check BEFORE you attempt to access a variable in an array that it is not equal to null.
Sunday, January 18, 2009
Dreams come true!!!
I have been recently hired as the Sun Campus Ambassador of KIIT-U, Bhubaneswar, India. Since the time I wrote my first Java program I was deeply affected by the simplicity of java yet its enormous potential, which slowly aroused my interest in knowing other Technology solutions from SUN.This growing interest led me to study javaSE, javaEE, javaME etc. and successful completion of several projects based on these technologies.
I felt overjoyed when I learnt that I have been selected as the next Campus Ambassador for my college, as now I can see a whole lot of opportunities to shape up my dreams about knowing the technology closely, have interaction with experts from round the globe, make friends world-wide who share common interest, same passion and zeal within like mine, opportunity to get certified directly by SUN, and of course a closer outlook of the working of a technology giant firm in corporate sector.
Well appreciated and supported by friends and mates, I am making my way through materializing various activities for the betterment of students of our college by letting them know about the current cutting-edge technology and many other interesting activities.